Hashing and Hash Tables
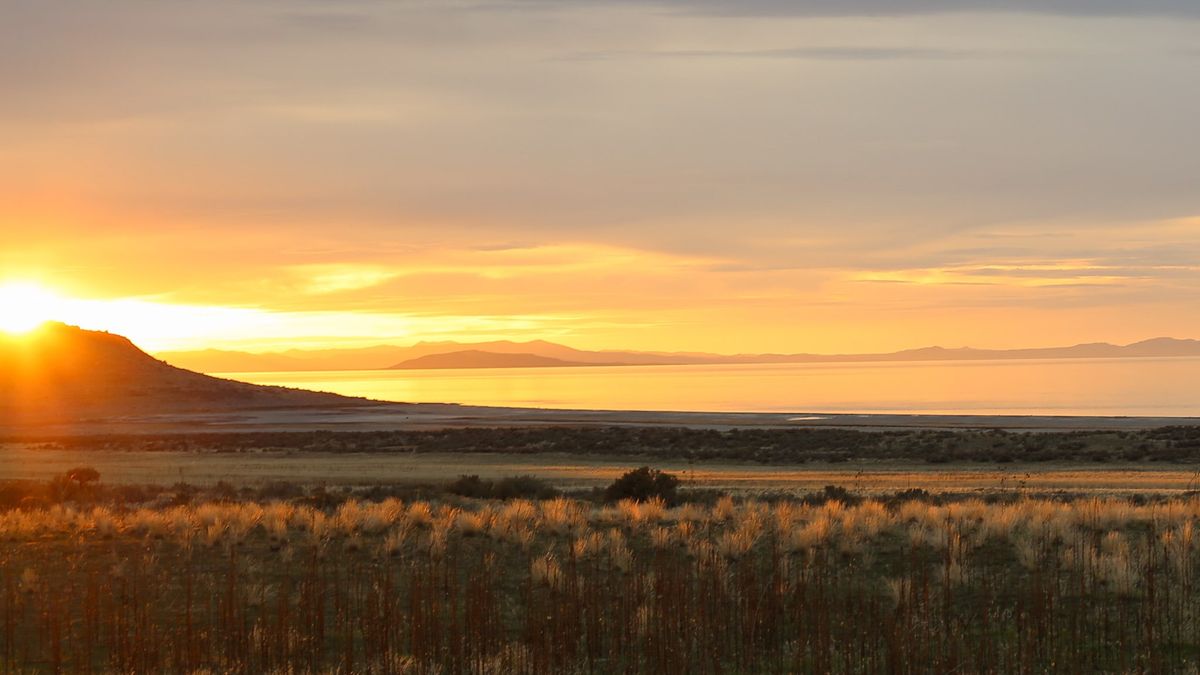
Today's topic is pretty straight forward. I got this one from the list of concepts that ChatGPT suggested. I think on days when I'm short on time or feeling less inspired, the generated list could be useful.
Sidebar: today I am feeling a bit underpowered because I went to get my blood drawn. More on this in the future as I find out more information.
What are hash tables?
A hash table is data type that uses keys and values to create associative arrays or in some programming languages it's known as a dictionary or hash map. The important difference between a basic associative array and a hash table is the hashing function which makes the lookup times much faster than if you used a generic array and created an associative array from it.
Python is one language that I know has a dictionary implemented in the standard library, I'm sure there are many others that do it too. Below is an example of what that should look like:
coordinates = {"latitude": "45.523064", "longitude": "-122.676483"}
print(f"Portland is located at {coordinates['latitude']} × {coordinates['longitude']}")
In this example, I'm using a simple lookup to get the latitude and longitudes of the coordinates
dictionary.
What is hashing?
Hashing is the important part that makes a hash table good as a data structure. A hashing function is used to convert a given key within the hash table to a unique index. This indexing allows constant lookup times which means that the size of the hash table does not matter as long as there is enough space for indexing of the keys.
How is a hash table different than an associative array?
According to my internet searching, it seems like in most cases, they're the same. But the important terminology difference lies in which context they're being used in. An associative array is usually an abstract concept and can be implemented using a hash table or a regular array, one that does not have a built in associative functionality. The term "hash table" is used to refer to the data structure that includes a key-value relation and includes a hashing function, in the context of computer science it's used as a catch-all for all the various names programming languages use like Object (JS), dictionary (python), HashMap (java), Hash (ruby), map (go), std::collections::HashMap (rust).
Conclusion
The good thing about today's learnings was that now I know that when people refer to maps, hash tables, associative arrays, they're usually the same aspect and do not really differ much, mainly the naming and programming language are the major difference. I didn't know about the hashing function which is seems to be the magic in all hash tables.
Comments ()